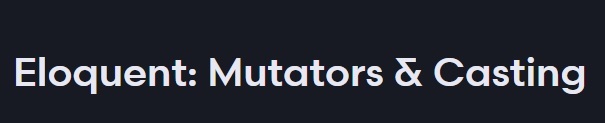
In my years of developing systems and API's for various business verticals, one of the most prominent problem I have encountered and still encountering until now are attributes that were not properly cast to their expected data type. It is always such a headache when you know you should be working with a `boolean` value but receiving a `string` instead. Or an `integer` or `float` value that is not properly mutated and left as `string`, especially when working on financial-related stuff. Or worse, an `array` of objects that is, guess what, left as `string`. In Laravel, attribute casting has vastly improved since version 7.x. We only used to have [Mutators and Accessors](https://laravel.com/docs/6.x/eloquent-mutators#array-and-json-casting) available to us but now, [class-based Custom Casts](https://laravel.com/docs/10.x/eloquent-mutators#custom-casts/) is available. And it is also rich with other sub-features such as [Value Object Casting](https://laravel.com/docs/10.x/eloquent-mutators#value-object-casting) and [Serialization](https://laravel.com/docs/10.x/eloquent-mutators#array-json-serialization). Just another reason to already bunch of reasons why I love working with Eloquent ORM. Casting is our first layer of assurance that we are getting correct data types post database query. And these framework-native features give us more than enough reason to always casts our attributes. So please, don't be lazy and utilize it, for your own sanity. **TIP:** If you don't use Eloquent when querying data from your database but still want your attributes to be cast properly? Just manually instantiate your rows instead: ``` use App\Models\Posts; $posts = \DB::table('posts')->get(); $posts = $posts->map(fn ($post) => new Post($post)); ```