In my years of developing systems and API's for various business verticals, one of the most prominent problem I have encountered and still encountering until now are attributes that were not properly cast to their expected data type.
It is always such a headache when you know you should be working with a `boolean` value but receiving a `string` instead. Or an `integer` or `float` value that is not properly mutated and left as `string`, especially when working on financial-related stuff. Or worse, an `array` of objects that is, guess what, left as `string`.
In Laravel, attribute casting has vastly improved since version 7.x. We only used to have [Mutators and Accessors](https://laravel.com/docs/6.x/eloquent-mutators#array-and-json-casting) available to us but now, [class-based Custom Casts](https://laravel.com/docs/10.x/eloquent-mutators#custom-casts/) is available. And it is also rich with other sub-features such as [Value Object Casting](https://laravel.com/docs/10.x/eloquent-mutators#value-object-casting) and [Serialization](https://laravel.com/docs/10.x/eloquent-mutators#array-json-serialization).
Just another reason to already bunch of reasons why I love working with Eloquent ORM.
Casting is our first layer of assurance that we are getting correct data types post database query. And these framework-native features give us more than enough reason to always casts our attributes.
So please, don't be lazy and utilize it, for your own sanity.
**TIP:** If you don't use Eloquent when querying data from your database but still want your attributes to be cast properly? Just manually instantiate your rows instead:
```
use App\Models\Posts;
$posts = \DB::table('posts')->get();
$posts = $posts->map(fn ($post) => new Post($post));
```
VueJS, InertiaJS, Laravel, TailwindCSS - more commonly known as **VILT** stack is one of the modern approaches for developing web apps with monolithic architecture.
In this post, I want to share with you some tips based on my personal experience developing with VILT stack.
# Inertia Version
When you install InertiaJS for Laravel, the process will need you to publish the middleware `HandleInertiaRequests` within your web middleware group. In this class is a public method `version` that is used by Inertia to determine the current version of your assets.
However, this particular method has some issues when you are running tests that are doing json assertions. What I recommend is to disable this when running tests, like so...
```
// app/Http/Middleware/HandleInertiaRequests.php
public function version(Request $request): ?string
{
return (! app()->runningUnitTests()) ? parent::version($request) : null;
}
```
# The Inertia Testing Header
[InertiaJS comes with its own tools and approach for writing tests and performing assertions.](https://inertiajs.com/testing) However, for endpoint tests, if you still want to use the traditional response assertions from Laravel, you can pass `['X-Inertia' => 'true']` as a header for each request like so...
```
public function can_get_user_edit_page()
{
$user = User::factory()->create();
$response = $this->get(
route('admin.user.edit', $user),
['X-Inertia' => 'true'] // Set this...
);
$response->assertJsonFragment(['component' => 'Admin/User/Edit'])
->assertJsonFragment(['name' => $user->name]);
}
```
# When Login is not an Inertia page
When developing an app that has a login page that is not part of Inertia pages, you will encounter this particular gotcha. When the authentication of your user expires, you will see your login page displayed on the modal like this:
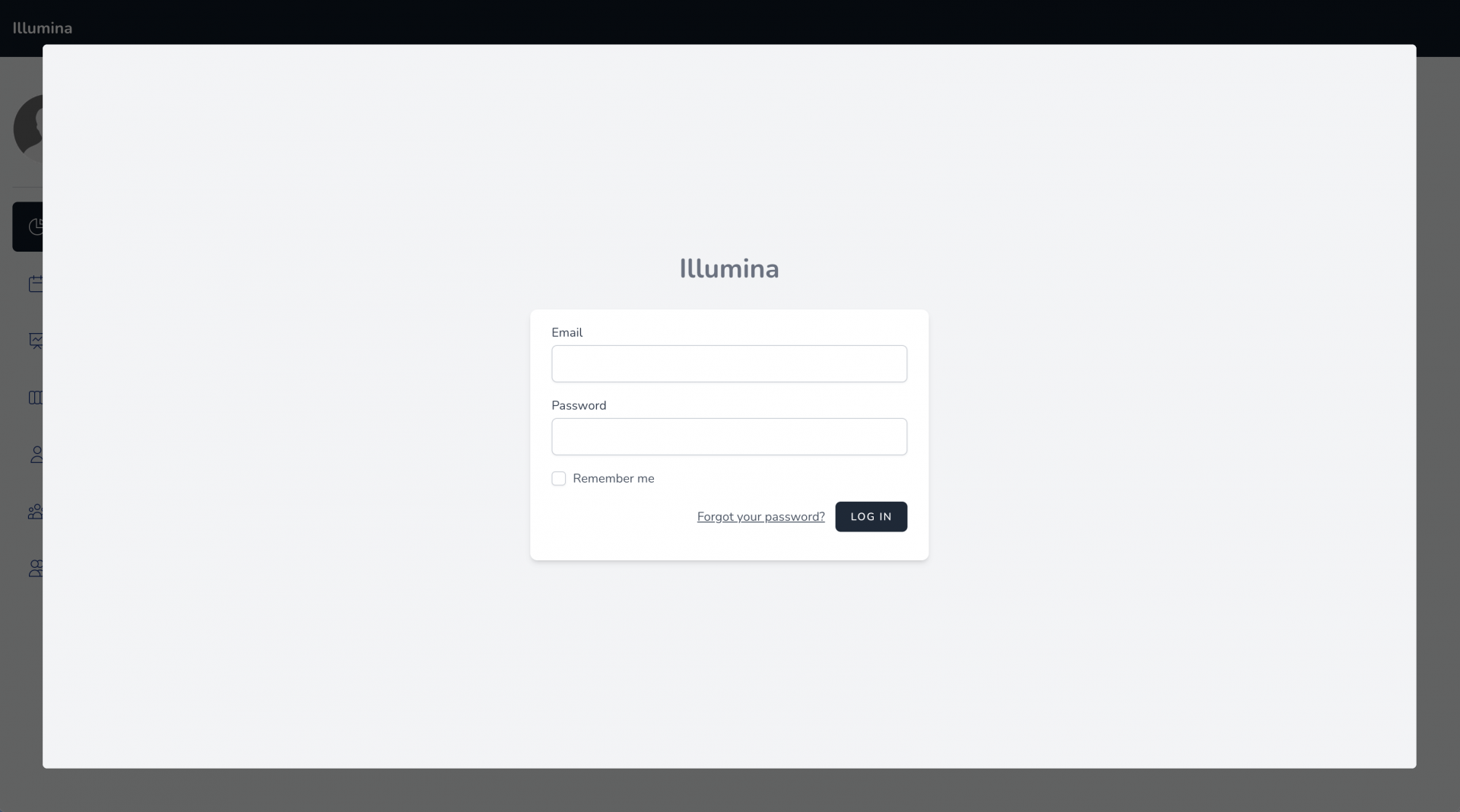
This would normally occur if the user left the app open and idle for quite sometime and their session expired, then they tried to navigate on an Inertia page. Manually refreshing the page would do the trick. But if the user tries to login through the form that is on the modal and is successful, it would then render the page on the modal. Pretty nasty UI issue.
You can easily replicate this by logging in a user, delete the session then navigate to an Inertia page.
Luckily, this can easily be solved by just overriding the `unauthenticated` method in your `App\Exceptions\Handler` class, and making it so that Inertia handles the redirection to the login page for HTTP response like so...
```
// app/Exceptions/Handler.php
use Inertia\Inertia;
class Handler extends ExceptionHandler
{
//...
protected function unauthenticated($request, AuthenticationException $exception)
{
// Check first if request is an inertia request.
// And if so, we redirect to the login page...
if ($request->hasHeader('X-Inertia', true)) {
return Inertia::location(route('login'));
}
if ($this->shouldReturnJson($request, $exception)) {
return response()->json(['message' => $exception->getMessage()], 401)
}
return redirect()->guest($exception->redirectTo() ?? route('login')
}
}
```
# Let TailwindCSS scan your PHP files
InertiaJS was made for building modern monolithic apps. This means coupling your frontend with your backend much like when building using Blade views. So there are times that some frontend entities like classes can come from the backend. For one, I like using Enums for determining status of certain models. The Enums would also hold some color values that frontend can render.
With that in mind, if you're using TailwindCSS as your frontend CSS framework, you might want to allow it to scan your PHP files so it can detect classes that are stored at the backend. You can do this by modifying your `tailwind.config.js` file and adding the app path within the content array, like so...
```
module.exports = {
content: [
'./resources/**/*.js',
'./resources/**/*.vue',
'./app/**/*.php',
],
}
```
# Inertia View Composer Package
This one would sound like a shameless plug. But one really powerful feature that InertiaJS has not yet implemented as of this writing is the ability to be able to share data to the frontend based on the page name. Laravel Blade views has this and its called [**View Composers**](https://laravel.com/docs/9.x/views#view-composers).
So you might want to check out this package that I created called [Kinetic](https://github.com/ambengers/kinetic) that does exactly that - share data to your frontend based on the Inertia page name. I've personally used this in one of the latest projects that I am working on and it helped me alot.
Happy coding!
Here are some straight-forward and practical tips on how to make your PHP code beautiful.
# Give it some space
As romantically-cliche as it may sound, sometimes all your code needs is a bit of space. A little bit of breathing room so you and your team can easily read it better. And as basic as it may seem, many, both new and experienced developers tend to still forget the idea of adding spaces on their code.
Take a look at these code blocks below...
```
public function test_can_upload_avatar()
{
$this->user = User::factory()->create();
$avatar = UploadedFile::fake()->image($filename = 'avatar.jpeg');
$this->post(route('user.profile.avatar'), ['avatar' => $avatar]);
$this->assertDatabaseHas('media', [
'model_type' => $this->user->getMorphClass(),
'model_id' => $this->user->id,
'file_name' => $avatar->hashName(),
]);
$this->assertNotNull($this->user->avatar->first());
$this->assertInstanceOf(Media::class, $this->user->avatar->first());
}
```
```
public function test_can_upload_avatar()
{
$this->user = User::factory()->create();
$avatar = UploadedFile::fake()->image($filename = 'avatar.jpeg');
$this->post(route('user.profile.avatar'), ['avatar' => $avatar]);
$this->assertDatabaseHas('media', [
'model_type' => $this->user->getMorphClass(),
'model_id' => $this->user->id,
'file_name' => $avatar->hashName(),
]);
$this->assertNotNull($this->user->avatar->first());
$this->assertInstanceOf(Media::class, $this->user->avatar->first());
}
```
They are obviously the same piece of code. I don't know about you, but just eye-balling these code blocks, I definitely find the latter much easier to take in and understand because of its ample spacing. Like, I don't feel the need to bring my face closer to the screen of my laptop just to be able to understand what each line of code does, if you know what I mean.
As a rule of thumb, try to put each statement (anything that ends with a semi-colon) on its own line unless 2 or more lines are doing the same type of action (i.e. the assertions on the example above, assigning variables, etc.).
# Use PHP CS Fixer
One advantage PHP has over other programming languages is that we have our own set of coding standards that developers generally agree upon. The [PHP Standards Recommendation or PSR](https://www.php-fig.org/psr/) are set of recommendations for styling our code. From **Basic Coding Standard**, **Coding Style** and **Autoloading Standards** the PSR has you covered so you don't have to invent your own.
Another good thing is that you can automate the implementation of these standards on your IDE using the [PHP-CS-Fixer](https://github.com/FriendsOfPHP/PHP-CS-Fixer) tool. Modern IDEs like Sublime Text, PhpStorm and VS Code have their own packages that you can just download from their respective package managers to set this up. All you need to do is to configure the set of rules that may want to implement for you or your team.
If you have no idea on what rules to implement, here's a link to a [gist of set of rules that I personally use in my projects](https://gist.github.com/ambengers/c112d75bb7e14b41daf99dc2abad7690). I have mine set to auto run whenever I save the PHP file so that I am sure that these standards will always apply no matter what.
You can also refer to this [PHP-CS-Fixer Cheat Sheet](https://mlocati.github.io/php-cs-fixer-configurator/#version:3.8) if you want to check on the meaning and effect of each rule.
# Leave Meaningful Comments
Another very basic yet many-tend-to-forget way of beautifying their code and helping themselves and/or their teammates understand their code better is by using comments. Not just comments, meaningful comments. Comments that actually makes sense when we you read it.
I personally believe that writing meaningful comments actually reflects how much care a developer have put into their code. This is actually one reason why Laravel framework is so popular. And like-minded developers tend to gravitate towards it, developers who actually care for their code.
Personally, as a rule of thumb, I leave comments on areas that I feel like, **if I don't remember the full context of how the code works, it will be quite hard for me to understand it again if I read it 6 months from the time that I wrote it**. I also try to follow Laravel's way of writing comments - where each line is a couple of characters shorter the the one above it.
Once in a while when diving deep into the Laravel codebase, I find that there are hidden gems that I think would really be helpful to developers. In this post, I'll shed light on one of those hidden gems.
I'm talking about the `AssertableJsonString::class` that is new since Laravel version 8.x. Let's go!
Typically when testing json responses, we have the ability to chain json assertions from the response like so:
```
public function test_json_response()
{
$response = $this->get(route('some.json.endpoint'));
$response->assertJson(['foo' => 'bar'])
->assertJsonMissing(['bar' => 'baz']);
}
```
Thanks to the fact that response is an instance of `Illuminate\Testing\TestResponse::class` which holds these convenient json assertion methods. However, there are **cases that we need to make assertions against json data that isn't coming from a json endpoint**. One example is reading a json file:
```
public function test_read_package_json_data()
{
$data = json_decode(
file_get_contents(base_path('package.json')),
true
);
// This won't work since $data is just a plain array and
// not an instance of Illuminate\Testing\TestResponse
$data->assertJsonFragment(["vue" => "^3.2.31"]);
}
```
Our typical assertions will not work on this example since `$data` is just a plain array. Before Laravel 8.x my workaround for this is to either pull in a third-party package specifically for json assertions, or wrap the json data with `Illuminate\Testing\TestResponse::class` like so:
```
use Illuminate\Http\Response;
use Illuminate\Testing\TestResponse;
...
public function test_read_package_json_data()
{
$data = json_decode(
file_get_contents(base_path('package.json')),
true
);
// Wrap the data with Illuminate\Testing\TestResponse class
// so that you can perform your typical json assertions...
$response = TestResponse::fromBaseResponse(new Response($data));
$response->assertJsonFragment(["vue" => "^3.2.31"]);
}
```
This approach works, but feels kind of hacky. For one, the data is not really an HTTP response so why does it have to go through these response classes?
Thankfully, Laravel 8.x extracted these json assertions into a class called `AssertableJsonString::class`!
Now we can just wrap our json data with this class and perform our assertions like so:
```
use Illuminate\Testing\AssertableJsonString
...
public function test_read_package_json_data()
{
$data = json_decode(
file_get_contents(base_path('package.json')),
true
);
$data = new AssertableJsonString($data);
$data->assertFragment(["vue" => "^3.2.31"])
->assertMissing(["php" => "^8.1" ]);
}
```
Enjoy coding!
In this post, I am going to talk about my personal opinion regarding DRY, KISS and YAGNI versus Coding Standards especially when working in a team setting.
I understand that you might not agree on some (or maybe even all) of the things that I have written here and I respect that. Everyone is entitled to their own opinion. So I encourage you all my readers, to look at this article with an objective perspective and a broader-than-usual mindset.
So with the disclosure out of the way, let me get to the point.
Always prioritize DRY, KISS and YAGNI over Coding Standards - even if you are in a team setting.
This comes with one condition however, that you need to have a good amount of test coverage so you have confidence in case that you need to refactor your code in the future.
**DRY *(Don't Repeat Yourself)*** - means that each small pieces of knowledge (code) may only occur exactly once in the entire system
**KISS *(Keep It Simple, Stupid!)*** - means to try to keep each small piece of software simple and unnecessary complexity should be avoided to not incur any technical debt.
**YAGNI *(You Ain't Gonna Need It!)*** - means that always implement things when you actually need them and never implement things before you need them.
I personally think these principles trump any coding standards a team might implement. And I am willing to go above and beyond that and say **DRY, KISS and YAGNI should be the guiding principles of teams when implementing Coding Standards**.
## Story Time!
I have recently started working as a Lead Developer in a team, and one of their Coding Standard is to **always use a FormRequest class** in the controller.
So here I am, working on a feature and I have one endpoint that only receives one parameter on the request, which is already covered with written tests. Also, mind you that this request parameter is only used for this endpoint and not anywhere else in the application.
```
public function update(Request $request)
{
$request->validate(['processed' => 'required|boolean' ]);
// Some other process here then return a json response...
}
```
Now, I am being told to refactor this into a `FormRequest` class. So I asked, why? Because I honestly don't see the need to. Why do I have to incur additional tech debt by adding a separate class that will only be used for validating a single request attribute? And this piece is not going to be used in any other place,so i'm not violating code duplication. What gives? KISS and YAGNI!
***But we have to follow Coding Standards!***
This is a classic example of letting the developers incur additional techical debt without any benefit at all, just for the sake of Coding Standards. In my most honest opinion, when the code is well-tested, readable and at its simplest form; when it can be easily changed when the need arise, then that is a good code.
And I understand the need to be one with the team you are working with, but programming is not a military industry but a creative one. And the possible amount of incurred technical debt when blindly following coding standards far outweighs that argument. And most of the time, that incurred technical debt is never paid. I have seen it multiple times.
Also, developers grow when they get to figure out their ways of deciding when to use certain methodologies and tools, and when to keep things simple. That decision can then be guided by the team during a code review session. And again, as long as the code is covered with tests, refactoring should never be a big concern.
As a developer, we often times deal with massive amount of nested relations and multi-dimensional arrays. Which also means from time to time we get hounded by some unexpected `Undefined index` or `Trying to get property of a non-object` errors.
For me, I often times experience this when I'm working on generating excel reports. So let me give you some real world (almost) examples..
## Situation...
Let's say we are creating an export excel report functionality for a helpdesk ticketing app.
We have a `$ticket` model that has an `id` field. It belongs to an `$approver` model that has a `full_name` field. So when we plot on excel sheet we want to know who is the approver of the ticket.
Somewhere in our code we will have something like:
```
// Assume that the array keys are the excel headings..
return [
'ticket_id' => $ticket->id,
'approver' => $ticket->approver->full_name,
];
```
At first this looks all good. However, if the **ticket has not yet been approved** `$ticket->approver` will result to `null`. And since you are basically doing `null->full_name` you'll get the really nasty error of `Trying to get property 'full_name' of non-object`.
In reality, if `$ticket->approver` is null, we want it to just return `null` so the excel cell can be empty and not worry about any error.
## optional(...) to the rescue!?
Yes! The magical `optional(...)` helper function can help here. So we can just say:
```
return [
'ticket_id' => $ticket->id,
'approver' => optional($ticket->approver)->full_name,
];
```
This looks quite okay already. You submit a PR and what a productive day it is, right?
But then your client decided to add another caveat. Now `$approver` model belongs to a `$company` model that has a `name` field in it, and your client wants that added on the excel sheet too.
No problem!
```
return [
'ticket_id' => $ticket->id,
'approver' => optional($ticket->approver)->full_name,
'approver_company' => optional($ticket->approver)->company->name,
];
```
However, if for some reason `$company` is not present from the approver model then we'd be back to that nasty error again. Okay so we can just wrap it in another `optional(...)` right? Well, we can do that... but it does not look clean... and there is a better solution.
## Arr::get(...)
If you are not yet familiar with what this helper does, take a look at the [official documentation here!](https://laravel.com/docs/8.x/helpers#method-array-get).
Let's go ahead and change our implementation:
```
use Illuminate\Support\Arr;
...
return [
'ticket_id' => Arr::get($ticket, 'id'), // Apply here as well so it looks uniform :)
'approver' => Arr::get($ticket, 'approver.full_name'),
'approver_company' => Arr::get($ticket, 'approver.company.name'),
];
```
Now if either `$approver` or `$company` model is not set, we do not have to worry about the nasty error. And it works seamlessly on multi-level nesting too.
Note: Obviously, the implementation will not work if the inner relation is a `Collection`. That's a totally different approach when plotting on an excel cell.
Today at work I implemented Parallel Testing to one of our projects. Perfect timing as we also just recently upgraded to Laravel 8 (coming from Laravel 7).
I was certain that Parallel Testing is available in Laravel 8 as I followed the development of this feature since the beginning of this year. However, after installing `brianium/paratest` package to our app and running the command `php artisan test --parallel` I encountered this error:

This got me confused, so I checked the Laravel 8 documentation for Parallel Testing and it was there.
[https://laravel.com/docs/8.x/testing#running-tests-in-parallel](https://laravel.com/docs/8.x/testing#running-tests-in-parallel)
However, this doesn't solve the issue as nothing in the documentation mentions this error.
So I decided to dig a bit further. And as it turns out, Parallel Testing was originally created for Laravel 9 and then backported to Laravel 8. Due to this, earlier versions of `nunomaduro/collision` package (5.0, 5.1, 5.2 - which what I was using at the time) had a restriction to only use `--parallel` option on Laravel 9, but version 5.3 removed that restriction.
[This link shows comparison of collison version 5.2 to 5.3.](https://github.com/nunomaduro/collision/compare/v5.2.0...v5.3.0) Checkout line 74 of `src/Adapters/Laravel/Commands/TestCommand.php` file for the removal of restriction.
So I promptly updated `nunomaduro/collision` to `^5.3` on my project and fixed the issue.
While at it I also took the liberty of creating a [pull request to laravel documentation](https://github.com/laravel/docs/pull/7022) so that this issue is properly documented and other developers don't waste their time in the future.
Peace!